How to work with a next.js application
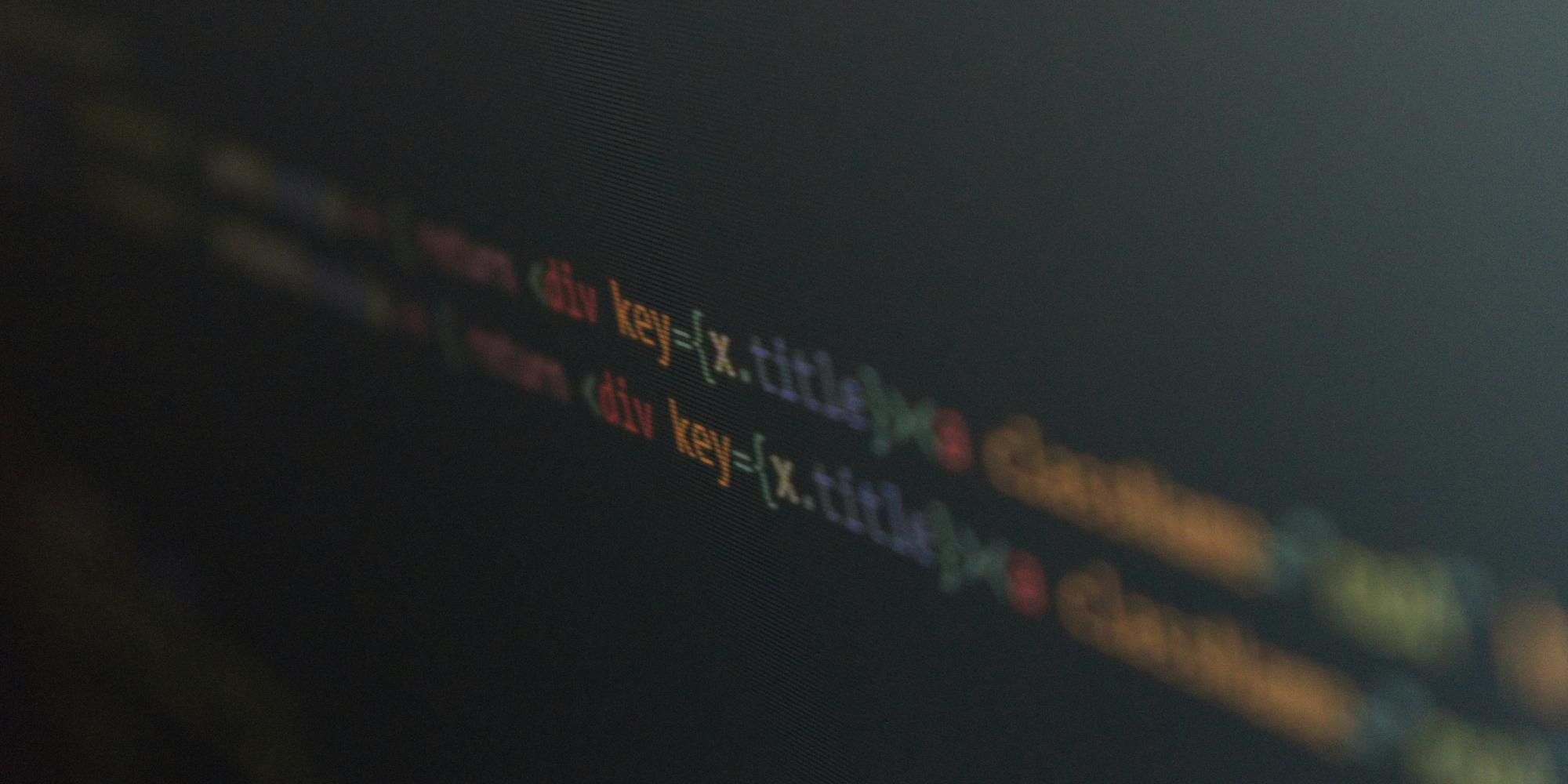
Prerequisites
Make sure you follewed the prerequisites from the previous post.
Writing a next.js application
A next.js application is a React application featuring server-side rendering, static site generation, and more. In this post, we will explore how to write a next.js application.
File structure
A next.js application has the following file structure:
my-next-js-app
├── src
│ └── app
└── public
The src
directory contains the source code of the next.js application. The public
directory contains static files like images, fonts, and more.
Since we have created our next.js application with the app-router
template, we have an app
directory inside the src
directory.
In this directory, we have the following files:
app
├── favicon.ico
├── globals.css
├── layout.tsx
└── page.tsx
The favicon.ico
file is the favicon of the next.js application.
The globals.css
file contains global styles for the next.js application.
The layout.tsx
file is the layout component of the next.js application.
The page.tsx
file is the main page component of the next.js application.
Creating a new page
To create a new page in the next.js application you need to create a new directory inside the src/app
directory.
For example, let's create a new page called about
:
mkdir src/app/about
The name of the directory determines the URL of the page. You can also add dynamic routes, but more on this later.
Now we can create our page inside the about
directory:
app
├── favicon.ico
├── globals.css
├── layout.tsx
├── page.tsx
└── about
└── page.tsx
The page.tsx
file inside the about
directory is the main component of the about
page.
You can now add your content to this file.
const AboutPage = () => {
return (
<div>
<h1>this is my about page</h1>
</div>
);
};
export default AboutPage;
Adding a link to the new page
To add a link to the new page we modify the src/app/layout.tsx
file. By this we ensure that the link is available on every page of the application.
We will use the Link
component from next/link
to create a link to the new page and one to return to the home page.
import type { Metadata } from "next";
import { Inter } from "next/font/google";
import "./globals.css";
import Link from "next/link";
const inter = Inter({ subsets: ["latin"] });
export const metadata: Metadata = {
title: "My Next.js App",
description: "My new Next.js app!",
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body className={inter.className}>
<div className="flex items-center justify-center space-x-4">
<Link href="/">Home</Link>
<Link href="/about">About</Link>
</div>
{children}
</body>
</html>
);
}
This allows us to navigate between about
and home
page.
Dynamic routes
Next.js allows you to create dynamic routes by adding square brackets []
to the name of a directory or file.
For example, let's create a dynamic route for a blog post. We create a new directory called [id]
inside the src/app
directory:
mkdir src/app/[id]
Now we can create our page inside the [id]
directory:
app
├── favicon.ico
├── globals.css
├── layout.tsx
├── page.tsx
├── blog
│ └── [id]
│ └── page.tsx
└── about
└── page.tsx
We give the created file page.tsx
the following content:
type Params = {
params: {
slug: string;
};
};
const BlogPage = ({ params }: Params) => {
return (
<div>
<h1>Hi, I am blog page {params.slug}!</h1>
</div>
);
};
export default BlogPage;
Now we can access the blog page with the URL http://localhost:3000/blog/my-first-blog-post
.
"use client"
By default every page is server-side rendered. If you want to use client-side rendering you can use "use client"
at top of the page.
"use client";
export default function MyComponent() {
const [state, setState] = useState(false);
return (
<div>
<button onClick={() => setState(!state)}>Toggle</button>
{state && <h1>Client-side rendered content</h1>}
</div>
);
}
This is needed for example if you want to use useState
or useEffect
.
Next steps
In this post, we have learned how to create a next.js application, how to create new pages and how to route between them. Furthermore, we have learned how to create dynamic routes in a next.js application. In the next post, we will learn how to deploy our next.js application using Docker.
Read Next
How to create a next.js application with create-next-app
In this post we will explore how to create a next.js application using create next app.
How to create a next.js blog application with mdx and static generation
Explore how to create a next.js blog application with markdown support and static generation at build time.
How to deploy a next.js application on docker hub
Explore how to deploy a next.js application on docker hub.